|
Page 1 of 1
|
[ 9 posts ] |
|
Another lua velocity error/question
Author |
Message |
Asatruer
Joined: Thu May 28, 2009 3:59 pm Posts: 209
|
 Another lua velocity error/question
Fist time really working with lua and with my essentially no experience with it I have run into what is probably a simple error, but I do not know how to fix it.  Here is the section of code where the error is coming from Code: function Update(self) if self.LTimer:IsPastSimMS(500) then if self.Vel.Magnitude < self.velMaximum then self.volocity = self.Vel.Magnitude + 50; self.Vel:SetMagnitude(self.volocity); end end end The code is attached to an MOSRotating that is a bullet that I am attempting to make start at a low velocity of 20 (set by FireVelocity in the Round) and speed up constantly after 500ms until it hits a maximum velocity.
|
Wed Jun 17, 2009 2:22 am |
|
 |
Kyred
Joined: Sun May 31, 2009 1:04 am Posts: 308
|
 Re: Another lua velocity error/question
Asatruer wrote: Fist time really working with lua and with my essentially no experience with it I have run into what is probably a simple error, but I do not know how to fix it.  Here is the section of code where the error is coming from Code: function Update(self) if self.LTimer:IsPastSimMS(500) then if self.Vel.Magnitude < self.velMaximum then self.volocity = self.Vel.Magnitude + 50; self.Vel:SetMagnitude(self.volocity); end end end The code is attached to an MOSRotating that is a bullet that I am attempting to make start at a low velocity of 20 (set by FireVelocity in the Round) and speed up constantly after 500ms until it hits a maximum velocity. There's actually an easier, and more efficient* way, to do this. You can have the bullet emit particles (invisible if you want them to be) out the back of the bullet and have these emissions push the bullet. Here's how it's done with the Ronin Fraction's Rocket Launcher: Code: EmissionAngle = Matrix AngleDegrees = 180 AddEmission = Emission EmittedParticle = MOPixel CopyOf = Jetpack Blast 1 BurstSize = 1 Spread = 0 MaxVelocity = 10 MinVelocity = 10 PushesEmitter = 1 *Pardon my lecture, but if your able to do something though a .ini mod, as apposed to a Lua script, it's better to take the .ini mod route. Anything defined in the .ini mod is handled directly by the engine code. Lua scripts, when run, are constantly being interpreted into engine code and then executed by the engine, making them more resource heavy.
However, since your script doesn't involved a lot of code, I don't think the extra resource tax will matter much, unless there will be like 60+ of these bullets on screen at once.However, if you prefer to do it in Lua, here's what's wrong with your script: self.Vel is defined by the game as a "const Vector," which from my experiences means vector functions don't work on them. You'll have to make a new vector, set it's magnitude with SetMagnitude(), and then add (since this time it's a vector) it to self.Vel. Code: local tempVel = Vector(0,0); self.Vel = self.Vel + tempVel:SetMagnitude(50);
|
Wed Jun 17, 2009 2:58 am |
|
 |
Asatruer
Joined: Thu May 28, 2009 3:59 pm Posts: 209
|
 Re: Another lua velocity error/question
Kyred wrote: You can have the bullet emit particles (invisible if you want them to be) out the back of the bullet and have these emissions push the bullet. Here's how it's done with the Ronin Fraction's Rocket Launcher: I have never noticed the Ronin 'zooka, or any of the vanilla launchers, accelerating after the initial shot, and that is not the effect I am going for. Kyred wrote: Pardon my lecture, but if your able to do something though a .ini mod, as apposed to a Lua script, it's better to take the .ini mod route. Anything defined in the .ini mod is handled directly by the engine code. I had actually been working on it for a long time as just an .ini edit trying to get various AEmitters to work based on p3lb0x's Dummy APRL, but could not get it to act in a good fashion. Good meaning hits and causes at least some damage at all ranges and is not reliant on gibbing into an explosion to cause that damage, and does not ricochetting off things and hitting the shooter... So I gave up to see if I could do it easier with lua. Kyred wrote: However, if you prefer to do it in Lua, here's what's wrong with your script: self.Vel is defined by the game as a "const Vector," which from my experiences means vector functions don't work on them. You'll have to make a new vector, set it's magnitude with SetMagnitude(), and then add (since this time it's a vector) it to self.Vel. Code: local tempVel = Vector(0,0); self.Vel = self.Vel + tempVel:SetMagnitude(50);
Thanks, spliced that into my code like so Code: function Update(self) if self.LTimer:IsPastSimMS(500) then if self.Vel.Magnitude < self.velMaximum then local tempVel = Vector(0,0); self.Vel = self.Vel + tempVel:SetMagnitude(50); self.Vel:SetMagnitude(self.volocity); end end end and I am no longer getting the error, but bullet does not appear to speed up at all. and I still get that original error and the bullets still always speed up and zip off the the right rather than in the direction it was fired in.
|
Wed Jun 17, 2009 3:29 am |
|
 |
piipu
Joined: Mon Jun 30, 2008 9:13 pm Posts: 499 Location: Finland
|
 Re: Another lua velocity error/question
Code: function Update(self) if self.Vel.Magnitude < self.velMaximum and self.LTime:IsPastSimMS(500) then self.Vel = Vector((self.Vel.Magnitude + 0.1) * math.cos(self.Vel.AbsRadAngle) , (self.Vel.Magnitude + 0.1) * math.sin(self.Vel.AbsRadAngle)) end end That will work unless there's some typoes. Also, you don't want to add 50 to the magnitude every frame.
|
Wed Jun 17, 2009 9:48 am |
|
 |
Asatruer
Joined: Thu May 28, 2009 3:59 pm Posts: 209
|
 Re: Another lua velocity error/question
Thanks piipu, that works a little bit better. It is now going left or right depending on which direction it is shot, but it has no vertical gain or drop, and is doing a strange gittery thing 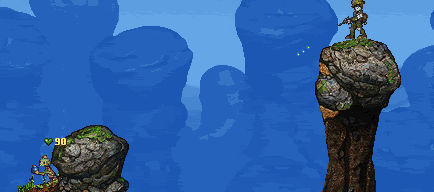 I have no idea what the code for it would look like, but would determining the direction of travel when it is created and trying to apply velocity increases to that work?
|
Wed Jun 17, 2009 4:39 pm |
|
 |
piipu
Joined: Mon Jun 30, 2008 9:13 pm Posts: 499 Location: Finland
|
 Re: Another lua velocity error/question
Code: self.Vel = Vector((self.Vel.Magnitude + 0.1) * math.cos(self.Vel.AbsRadAngle) , -(self.Vel.Magnitude + 0.1) * math.sin(self.Vel.AbsRadAngle)) Just negating the Y component should work. Replace the self.Vel = stuff line with that. Edit: Oops, forgot to actually negate it. Oh well, should work better now.
|
Wed Jun 17, 2009 4:43 pm |
|
 |
Asatruer
Joined: Thu May 28, 2009 3:59 pm Posts: 209
|
 Re: Another lua velocity error/question
Great, that work. Now to teak things so it accelerates as fast as I want it to, and I need to figure out how to deal with the initial drop due to low velocity.
|
Wed Jun 17, 2009 6:34 pm |
|
 |
mail2345
Joined: Tue Nov 06, 2007 6:58 am Posts: 2054
|
 Re: Another lua velocity error/question
GlobalAccScalar?
|
Wed Jun 17, 2009 7:09 pm |
|
 |
Asatruer
Joined: Thu May 28, 2009 3:59 pm Posts: 209
|
 Re: Another lua velocity error/question
mail2345 wrote: GlobalAccScalar? Bleah, yeah... that was it. Thanks for pointing out the obvious. Apparently when I scrapped all the AEmitters that emitted AEmitters like the DAPRL, I did not carry over the GlobalAccScalar into my new MOSRotating. It flies mostly straight now. Now I have to get it to actually cause damage.
|
Thu Jun 18, 2009 6:34 am |
|
 |
|
|
Page 1 of 1
|
[ 9 posts ] |
|
Who is online |
Users browsing this forum: No registered users |
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot post attachments in this forum
|
|